Overview
Cooking Papa is a desktop cookbook application used to manage recipes and ingredients for cooking. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 15 kLoC.
Summary of contributions
-
Major enhancement: Added the ability to cook a recipe using inventory ingredients
-
What it does: Allows the user to remove the ingredients in the inventory after cooking a specific recipe inside CookingPapa.
-
Justification: This feature improves the product significantly because it allows the user to remove recipe’s ingredients effectively without having the user to remove the each ingredient separately.
-
Highlights: This enhancement will be one of the most used feature as this application is mainly to aid cooking experience. This enhancement helps to integrate the
Cookbook
andInventory
systems together to provide a all-in-one application which make this enhancement challenging also. -
Credits: The implementation of the
Inventory
andCookbook
classes was a team effort which is vital to the successful implementation of this feature.
-
-
Minor enhancement:
-
Contributed to basic structure of
Step
andTag
classes in recipe. -
Contributed to basic
Recipe
command classes such as managing tag and steps in a recipe.
-
-
Code contributed: [by @hans555]
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Inventory Commands (by Ong Han Sheng)
Inventory commands are commands that update the user’s very own inventory at home. These commands include adding, remove and viewing the current inventory database.
Add an ingredient to the inventory
This commands allows you to add ingredients to your inventory. Ingredient names added are case-insensitive. Ingredient names such as 'Bacon' and 'bacon' will be recognised by CookingPapa as 'Bacon'.
-
Format:
inventory add ingredient i/INGREDIENT q/QUANTITY
-
Examples:
Command | Result |
---|---|
|
Adds 10 eggs into your inventory. |
|
Adds 200g of butter into your inventory. |
Remove an ingredient from the inventory
This command allows you to remove ingredients from the inventory. You can indicate the quantity you want to remove for an ingredient, which should not be greater that than the ingredient’s quantity. Please ensure that the unit of the quantity matches the ingredient’s unit in the inventory.
Note: If you do not indicate any quantity, all entries in the inventory that have the specified ingredient name will be removed regardless of the unit.
-
Format:
inventory remove ingredient i/INGREDIENT [q/QUANTITY]
-
Examples:
Command | Result |
---|---|
|
Removes all bacon from your inventory. |
|
Removes 200g of butter from your inventory. (If your inventory had 500 g of butter, it will be subtracted and updated to 300 g of butter) |
Remove all ingredients from the inventory.
This command allows you to remove all ingredients from the inventory.
-
Format:
inventory clear
-
Example:
Command | Result |
---|---|
|
Removes all ingredients from the inventory |
Cook a recipe using the ingredients in the inventory
This command allows you to remove the selected’s recipe ingredients from the inventory after cooking a delicious meal.
-
Format:
inventory cook recipe INDEX
-
Example:
Parameters | Result |
---|---|
|
Removes all ingredients required to cook recipe 3 from the inventory |
Recipe of the day generator [v2.0] (by Ong Han Sheng)
This command allows CookingPapa to recommend a recipe to cook based on the recipes currently in the cookbook.
-
Format:
generate recipe of the day
-
Example:
Command | Result |
---|---|
|
Displays the recipe of the day |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Add a Recipe’s Ingredients to Cart (by Ong Han Sheng)
The user may want to buy the required ingredients to cook a certain recipe in the cookbook. This feature allows the user to add a certain recipe’s required ingredients into the cart.
Implementation
The action of adding a recipe’s ingredients to cart mechanism is facilitated by CartAddRecipeIngredientCommand
, which
extends the CartAddCommand
abstract class. The format is as follows: cart add recipe INDEX
.
This command is implemented to ease the tedious process of having the user adding every single ingredient
to their cart when they want to purchase ingredients to cook a certain recipe. This provides convenience to users
that frequently use our application and such process like shopping for a certain recipe’s ingredient is
intuitive to users. Furthermore, this command creates interaction between the Cookbook
and Cart
which
helps to further integrate the application as an all-in-one application.
Below is a step by step sequence of what happens when a user enters this command:
-
The user enters the command
cart add recipe INDEX
in the command line input. -
CartAddRecipeIngredientParser
parses the user input and checks if the index provided is an integer. Note that the parser will throw aParseException
if the given index is not an integer.try { recipeIndex = ParserUtil.parseIndex(argMultimap.getPreamble()); } catch (ParseException pe) { throw new ParseException(String.format(MESSAGE_INVALID_RECIPE_DISPLAYED_INDEX, CartAddCommand.MESSAGE_USAGE), pe); }
-
The index is passed as a parameter for
CartAddRecipeIngredientCommand
which is returned toLogicManager
. -
LogicManager
callsCartAddRecipeIngredientCommand#execute()
which checks if the given index is a valid index of a recipe. Note that the command will throw aCommandException
if the given index is not valid.if (recipeIndex.getZeroBased() >= model.getCookbook().getRecipeList().size()) { throw new CommandException(String.format(MESSAGE_INVALID_RECIPE_DISPLAYED_INDEX, MESSAGE_USAGE)); }
-
If the index is valid, the selected recipe’s ingredients will be added accordingly. This is done through calling
Model#addCartIngredient()
, with each ingredient as the parameter. -
Model#addCartIngredient
callsCart#addIngredident()
which then adds the ingredient to the cart. If a certain ingredient exists in the cart, adding a ingredient to a cart will increase the quantity instead. Otherwise, a new instance of that ingredient will be added to the cart. After adding an ingredient, the cart will be updated withModel#updateFilteredCartIngredientList()
. -
A
CommandResult
with the successful text message is returned toLogicManager
and will be displayed to the user via the GUI to feedback to the user that the selected recipe’s ingredients has been successfully added to the cart.
The following activity diagram shows a possible flow of events for a user using this feature:
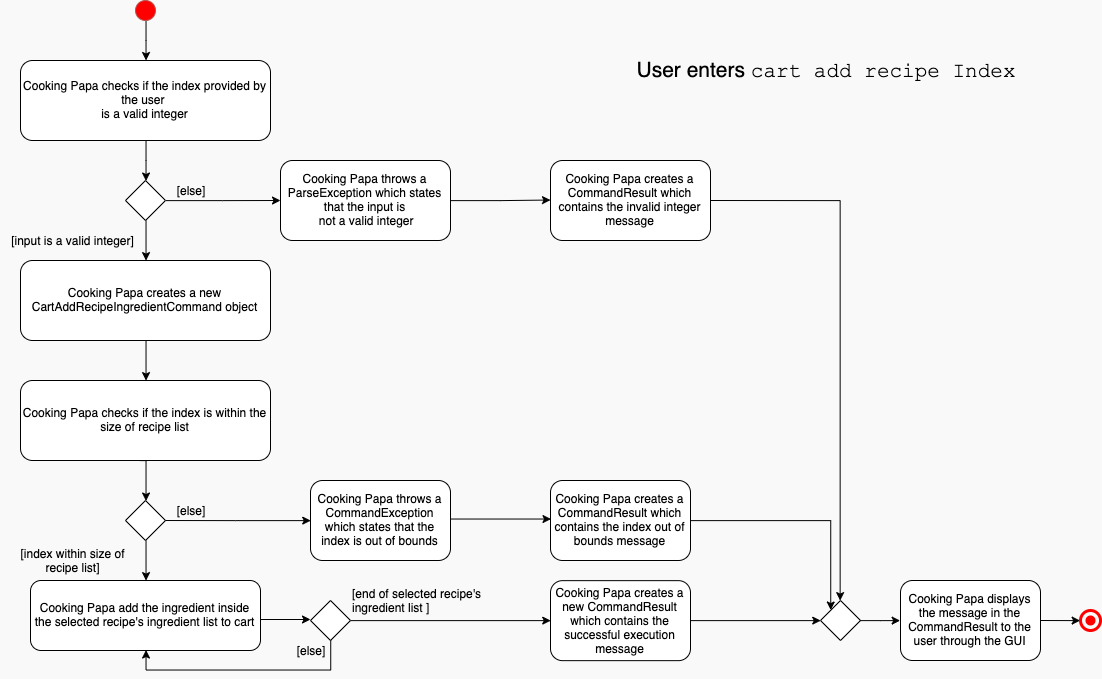
The following sequence diagram shows how the function of adding recipe’s ingredients to cart works:
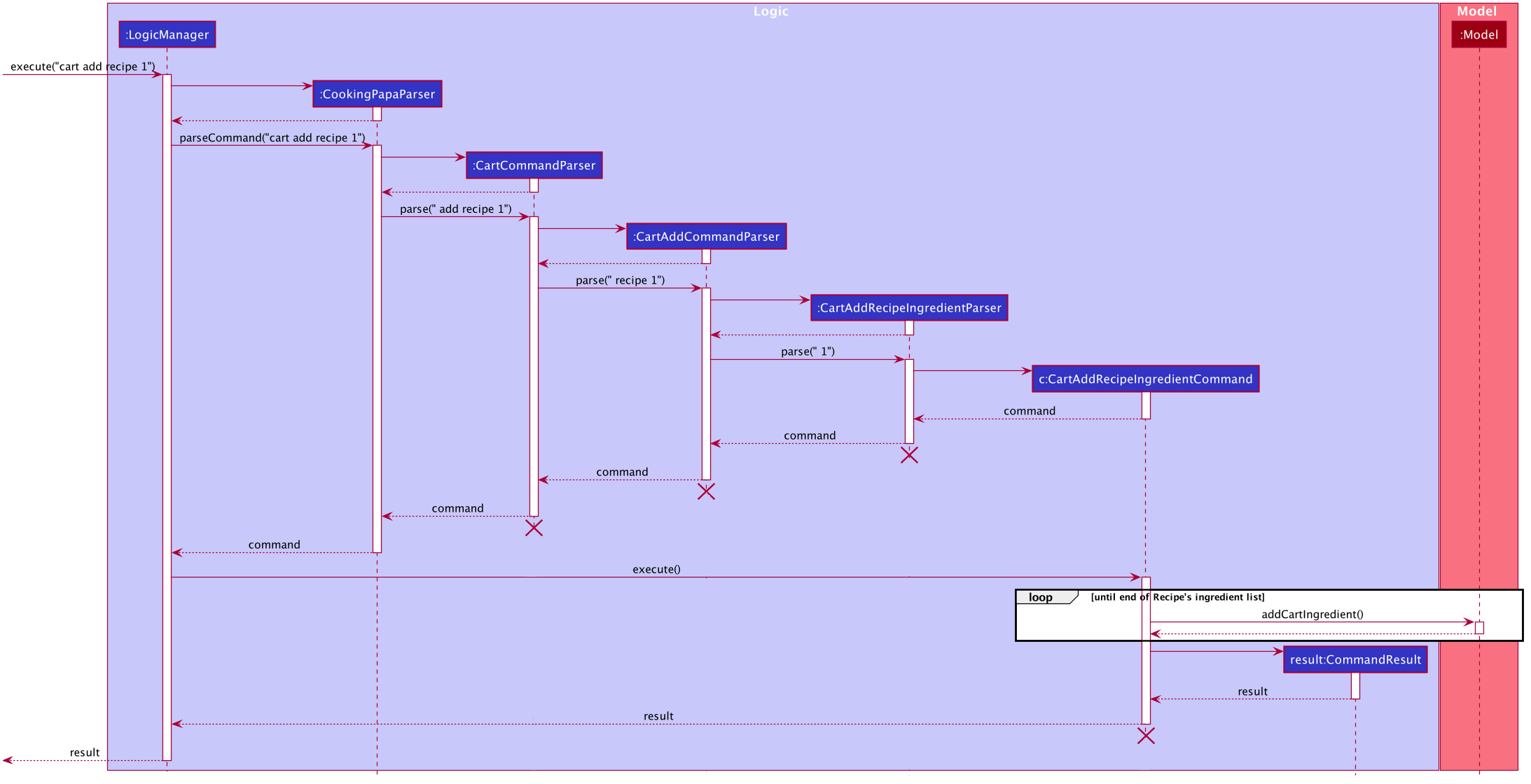
Design considerations
Aspect: Allowing users to add all or some recipe’s ingredients
Design A (current choice): Adding all recipe’s ingredients to the cart |
Design B: Adding only recipe’s ingredients that are missing in the inventory to the cart |
|
Description |
Allows user to add a recipe’s ingredients to the cart for shopping. This design is currently chosen due to ease of implementation and it works for all situations. |
Allows user to add a recipe’s ingredients base on the inventory status. However, there are some situations where this design not does work. One example would be like planning to cook at outside where the inventory status is unknown. |
Pros |
|
|
Cons |
|
|